This is the Building of GraphQL APIs that utilize Node
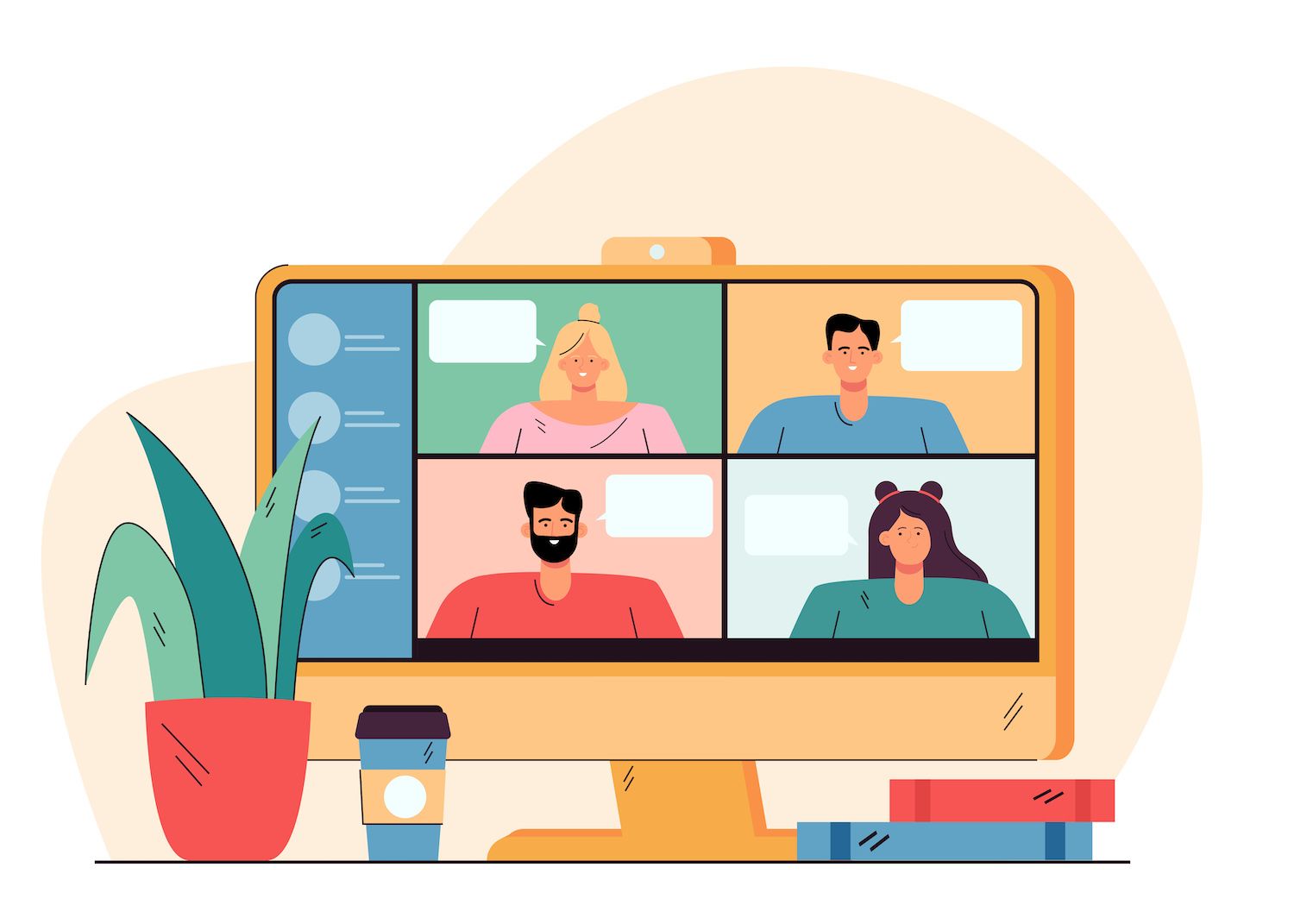
GraphQL is the most advanced technology utilized to build APIs. Although RESTful APIs are the method that is most often used to present information to applications but they do have drawbacks that GraphQL seeks to address.
GraphQL is the query language created by Facebook that was turned into an open source project in 2015. T provides a user-friendly and flexible syntax that can be used to describe and access information using an API.
What exactly is GraphQL?
As per the official website: "GraphQL is a API query language as well as an API runtime, which is able to be used in the completion of queries using your existing information. GraphQL offers a comprehensive and easy description of the data you offer through your API. It allows clients to get the information they want and not more. It makes it simple to create APIs in a short the time available as well as providing developers the ability to use robust tools."
To create a GraphQL service, begin with defining schema types before developing fields that are based on these types of. You then create the function resolver, which will run on each field and type whenever data needs to be gathered on the client's side.
The GraphQL Terminology
GraphQL type system is used to decide what data is able to be requested and the data that is possible to alter. This is the core of GraphQL. There are many ways to explain and change the information in the structure of GraphQ.
Types
GraphQL objects are objects that have fields that have a type of solid. It is recommended to make an 1-1 map between the model and GraphQL types. Here's an illustration GraphQL Type:
type User Type User's ID! "! "!" is the first name that is required. Stringlastname Email:: string username: String todos"[Todo]" a completely different GraphQL kind
Queries
GraphQL Query defines all the query queries that clients are able to use to access the GraphQL API. Clients should build the base querythat encompasses all currently running queries using the protocol.
We'll then identify the query and then match it to the RESTful API which is compatible with them:
Type RootQuery user(id ID) User # matches API/users/:idUsers: # is related to API/users todostodo(id: ID! ): Todo # Corresponds to GET /api/todos/:id todos: [Todo] # Corresponds to GET /api/todos
Mutations
In the event that GraphQL is one of the GET requests, then the changes include POST PUT, the PATCH, and the DELETE request that change the GraphQL API.
We'll combine all changes to create the one RootMutation to show:
type RootMutation *
It is possible that you have seen the usage of the -input types to define the types of transformations like the input from the user, TodoInput. It is always a good suggestion to identify input types before designing or altering the source.
It is possible to define Input kinds like this:
Input UserInput: Firstname String! Lastname String email String! username: String!
Resolvers
Create a brand new resolvers.js file and insert the following code in it:
import sequelize from '../models'; export default function resolvers () const models = sequelize.models; return // Resolvers for Queries RootQuery: user (root, id , context) return models.User.findById(id, context); , users (root, args, context) return models.User.findAll(, context); , User: todos (user) return user.getTodos(); , // Resolvers for Mutations RootMutation: createUser (root, input , context) return models.User.create(input, context); , updateUser (root, id, input , context) return models.User.update(input, ...context, where: id ); , removeUser (root, id , context) return models.User.destroy(input, ...context, where: id ); , // ... Resolvers for Todos go here ; }
Schema
GraphQL schema determines the information GraphQL provides to the public. Therefore, the types, queries, and mutations will be included in the schema and accessible to all users.
Here are steps on how to disclose types, questions and other changes that are made for the public to:
schema query: RootQuery mutation: RootMutation
The script that we have created above have added to it the RootQuery as and RootMutation which were previously developed to make them open to everyone in the world.
What is GraphQL Do? Work With Nodejs and Expressjs
GraphQL is a programming language that supports all programming languages and Node.js is not an one of them. This official GraphQL site has an article which covers JavaScript implementation and there are different versions of GraphQL that makes the program writing process with GraphQL effortless.
GraphQL Apollo provides an implementation for Node.js and Express.js and lets users get started with GraphQL.
The course will teach you how to create and build your first GraphQL application with Nodes.js and the Express.js backend framework that includes GraphQL Apollo in the following section.
Configuring GraphQL By using Express.js
Constructing a GraphQL API server using Express.js can be a great approach for novices to start. In this guide we'll cover how to build an GraphQL server.
Design a new Project with Express
Create a folder within your project, and then install Express.js via the following commands:
cd && npm init -y npm installation express
The command generates a new package.json file and it installs the Express.js library in your application.
Are you interested in learning how we've increased the amount of visitors we get by 1,000?
Join more than 20,000 others who receive our emails every week. It is packed with exclusive WordPress details!
We will then organize our work in the way depicted in the image below. The program will consist of a number of modules used in order to perform the functions of the application, such as user, task, etc.
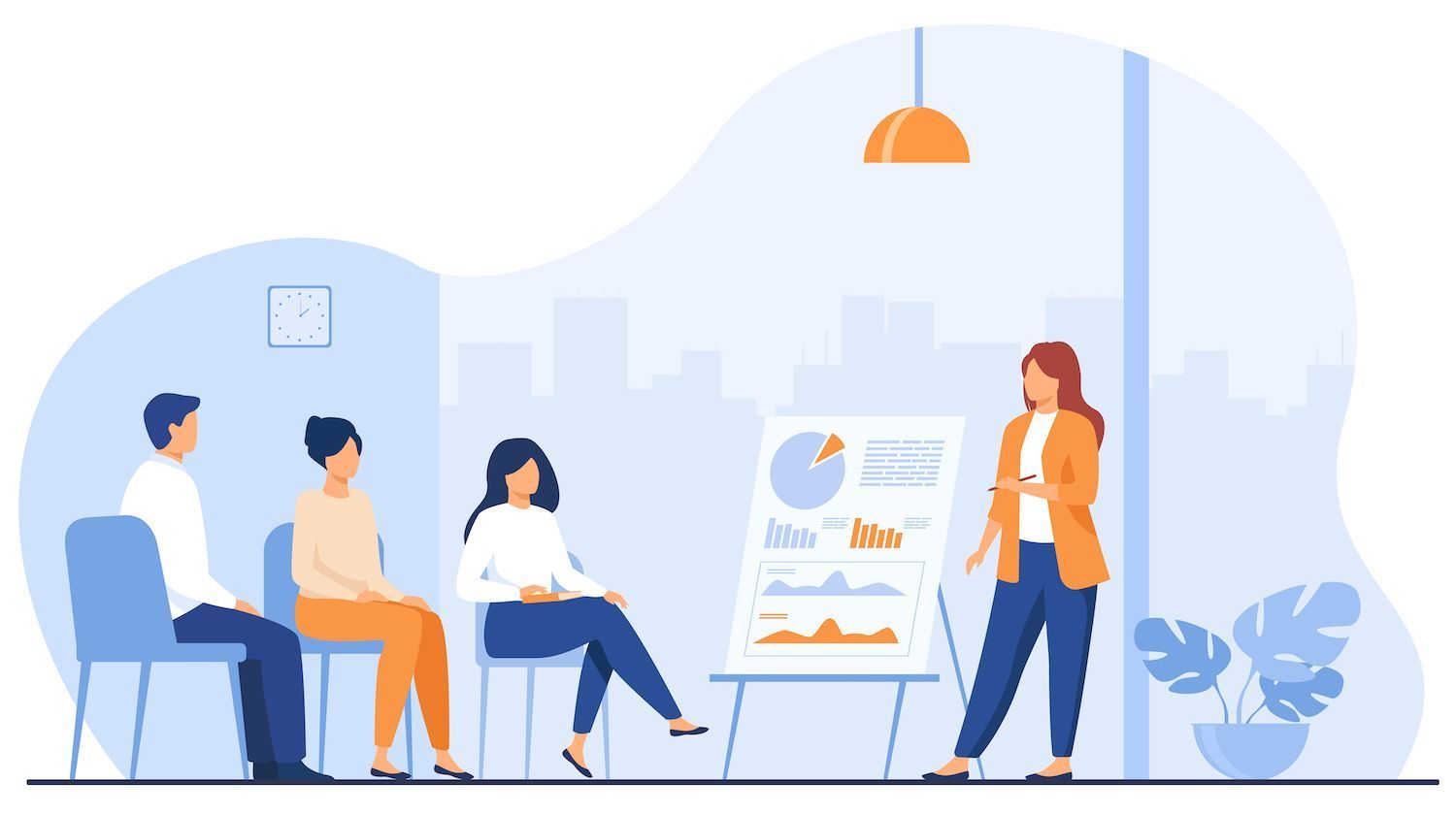
Initialize GraphQL
Set up your GraphQL Express.js dependency. The following command is needed to set up the installation
npm install apollo-server-express graphql @graphql-tools/schema --save
The method of making Schemas and Types
We will now create index.jsfile. index.jsfile inside the directory for modules. After that, we'll add the following code fragment:
const gql = require('apollo-server-express'); const users = require('./users'); const todos = require('./todos'); const GraphQLScalarType = require('graphql'); const makeExecutableSchema = require('@graphql-tools/schema'); const typeDefs = gql` scalar Time type Query getVersion: String! type Mutation version: String! `; const timeScalar = new GraphQLScalarType( name: 'Time', description: 'Time custom scalar type', serialize: (value) => value, ); const resolvers = Time: timeScalar, Query: getVersion: () => `v1`, , ; const schema = makeExecutableSchema( typeDefs: [typeDefs, users.typeDefs, todos.typeDefs], resolvers: [resolvers, users.resolvers, todos.resolvers], ); module.exports = schema;
Code Walkthrough
Let's look at this code-snippet to take it apart
Step 1.
After that, we download the needed libraries and set up the most common types that are query as well as mutation. Both queries and mutations are an instance of GraphQL API as of now. But, we plan to extend the query as well as the mutation to incorporate more schemas in the future.
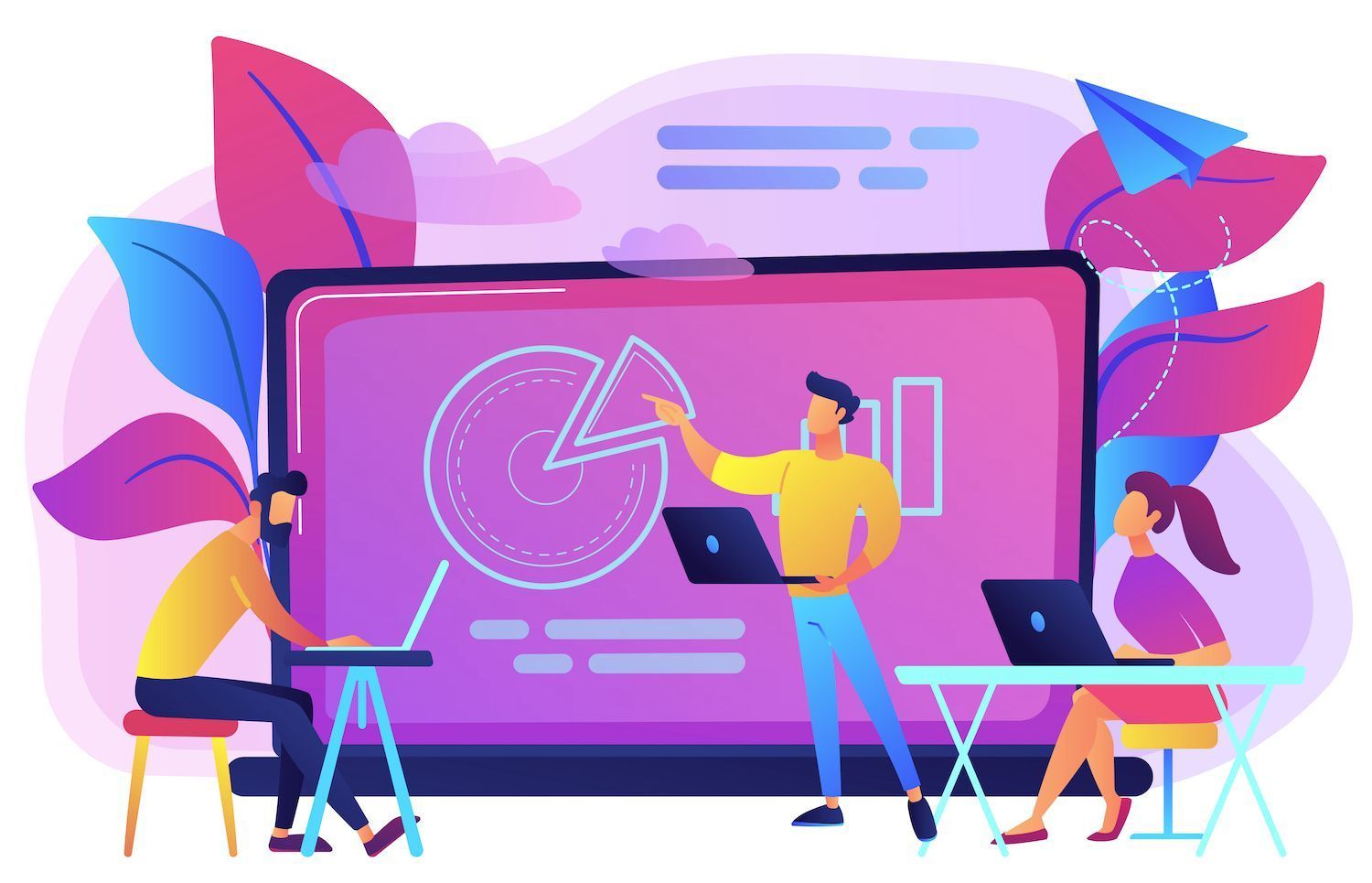
Step 2.
We then created the new kind of scalar which is a representation of time and also our first resolver for this query, as well as the transform that we had earlier. Additionally, we generated a schema using this createExecutableEchema function.
The schema created includes schemas that we have imported, and will add additional schemas in the future as we create and will later integrate these to the database.
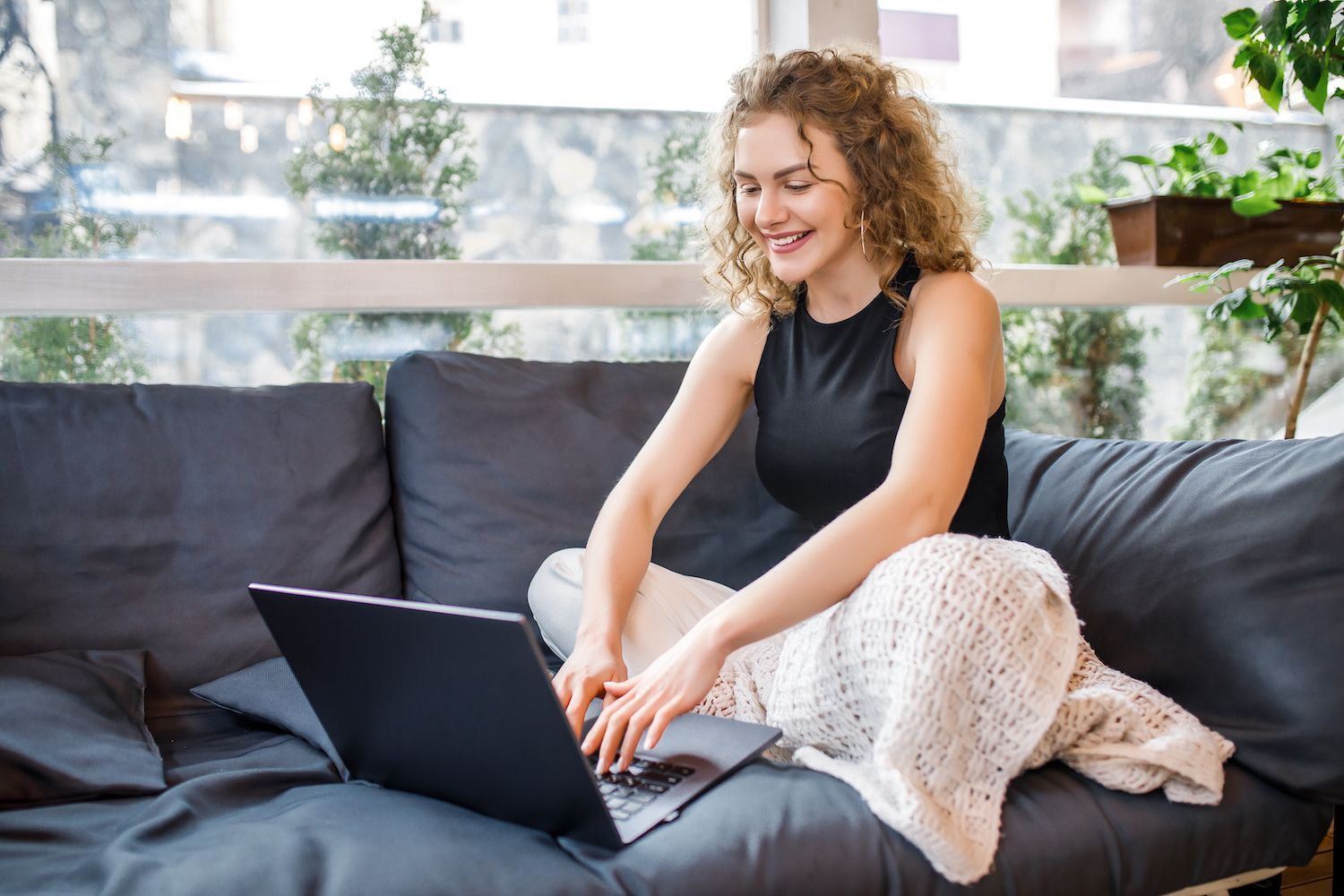
This example code shows how we have imported various schemas to the makeExecutableEchema method. This technique helps create an application that is well-organized to handle the complex issues. The next step is develop our own schemas that are based on the Todo and the User schemas that we have introduced.
Creating Todo Schema
The Todo schema describes the basic functions users of the app can perform. The following schema performs Todo operations , CRUD.
const gql = require('apollo-server-express'); const createTodo = require('./mutations/create-todo'); const updateTodo = require('./mutations/update-todo'); const removeTodo = require('./mutations/delete-todo'); const todo = require('./queries/todo'); const todos = require('./queries/todos'); const typeDefs = gql` type Todo id: ID! title: String description: String user: User input CreateTodoInput title: String! description: String isCompleted: Boolean input UpdateTodoInput title: String description: String isCompleted: Boolean *do; // Implement resolvers for the schema fieldsconst resolvers = Resolvers to resolve queriesQuery such as todo, todos and// Resolvers for Mutations Mutation: createTodo, updateTodo, removeTodo, , ; Module.exports = typeDefs;module.exports = typeDefs, resolvers ;
Code Walkthrough
Let's work through this code-snippet. We'll break it down:
Step 1.
After that, we created a structure to manage our tasks through the utilization the use of GraphQL form, input and expanding. The extension keyword extensions keyword lets you create additional queries or mutations within the base query as well as the mutation that the above-mentioned transformation has caused.
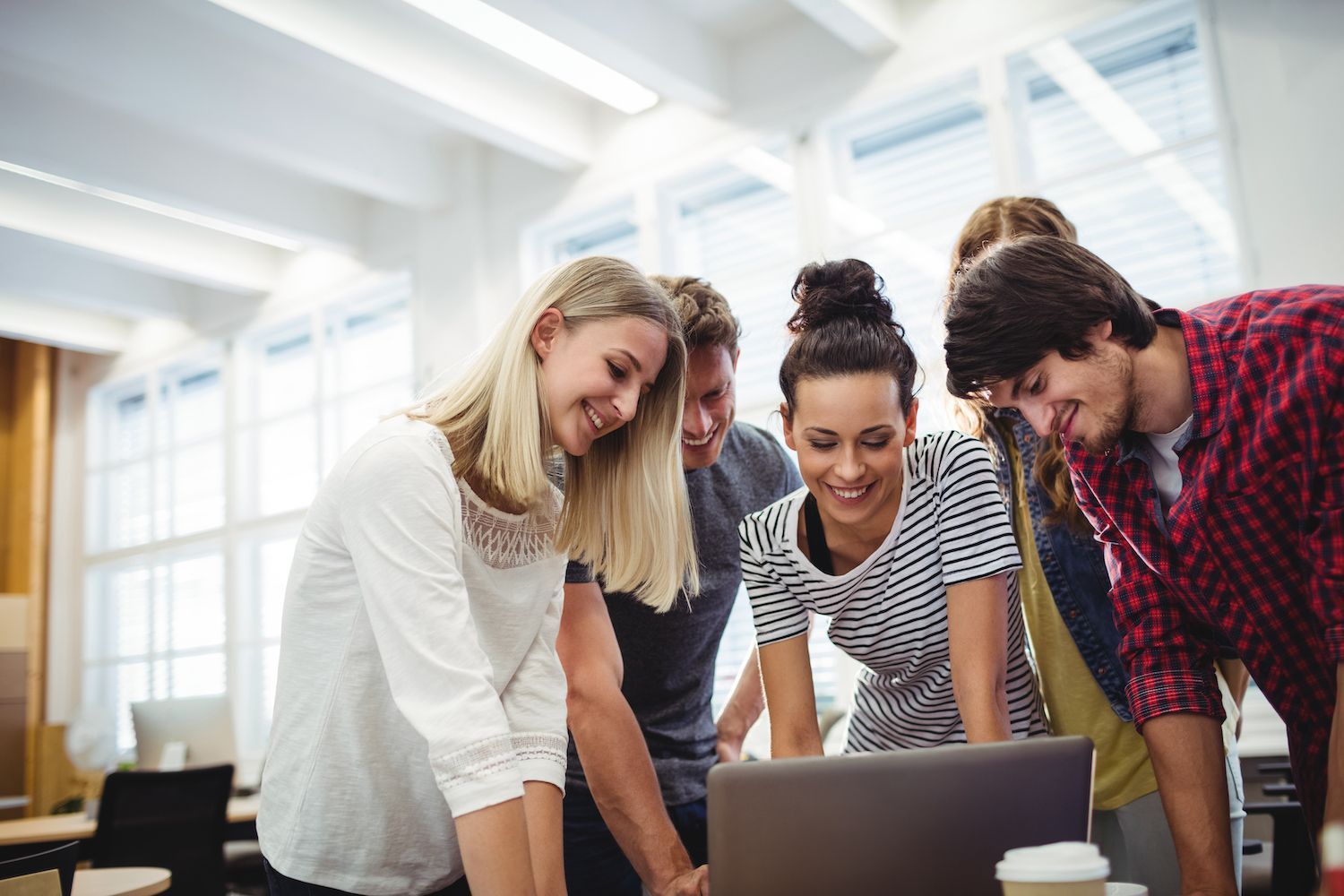
Step 2.
This is the resolver we created, which is able to locate the correct information when an issue or change is found to be.
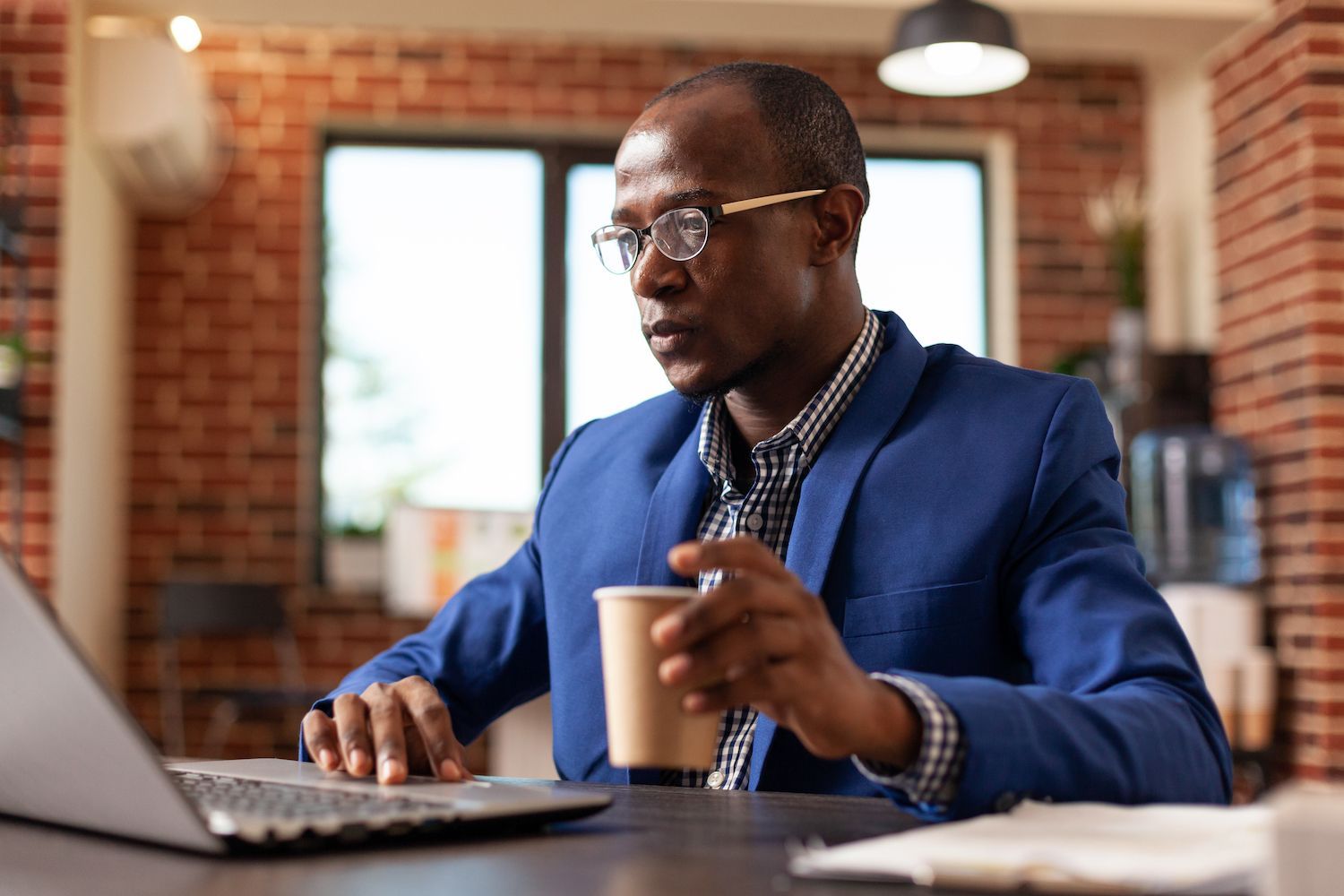
If we implement resolver functions, it's possible to create specific strategies to control the database as well as the business logic as in create-todo.js example.
Create a create-user.js file in the ./mutations
folder and integrate the business logic in order to create a brand new Todo in your database.
const models = require('../../../models'); module.exports = async (root, input , context) => return models.todos.push( ...input ); ;
The above code is a simplified way of creating the new Todo inside our database using an ORM Sequelize. You can learn more on Sequelize and how you can create it using Node.js.
It is possible to follow the same procedure to build diverse schemas dependent on the specifications of your application or you could transfer the entire application to GitHub.
The next step is to make a server using Express.js and to launch the latest Todo application by using GraphQL in conjunction with Node.js
Configure and then to run the Server
Lastly, we will set up our server using the apollo-server-express library we install earlier and configure it.
The apollo-server-express is a simple wrapper of Apollo Server for Express.js, It's recommended because it has been developed to fit in Express.js development.
Using the examples we discussed above, let's configure the Express.js server to work with the newly installed apollo-server-express.
Create the server.js file inside the root directory. Copy the server.js file in the root directory. Copy this code to it:
const express = require('express'); const ApolloServer = require('apollo-server-express'); const schema = require('./modules'); const app = express(); async function startServer() const server = new ApolloServer( schema ); await server.start(); server.applyMiddleware( app ); startServer(); app.listen( port: 3000 , () => console.log(`Server ready at http://localhost:3000`) );
The code you have seen above means that you've successfully completed the steps of creating your first CRUD GraphQL server for Todos and Users. You can start your development server and access the playground using http://localhost:3000/graphql. If everything goes as planned, you should see the following display that follows:
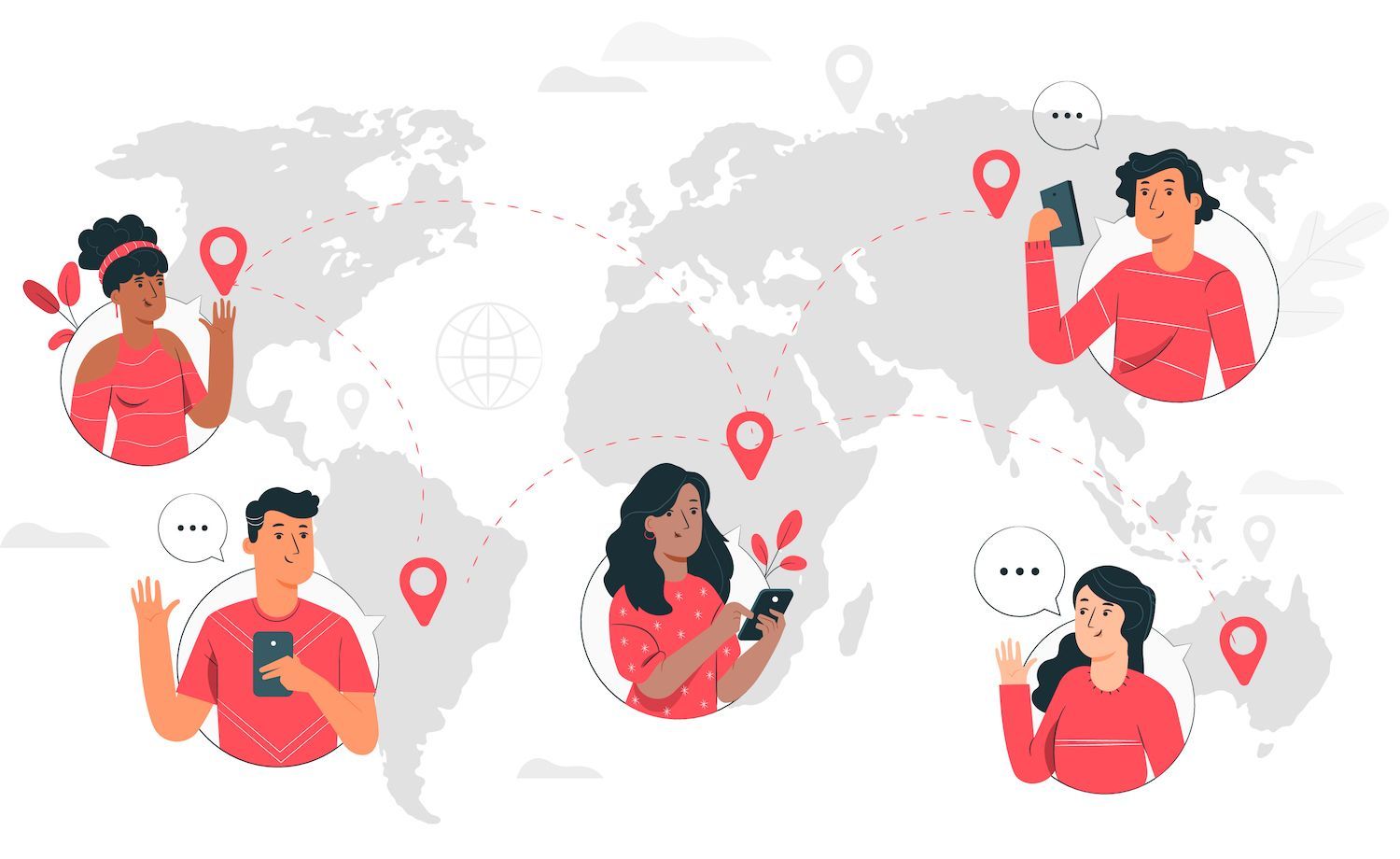
Summary
GraphQL is a modern-day technology utilized by Facebook that facilitates the task of creating vast-scale APIs using RESTful architectural designs.
This article has provided an overview of GraphQL and showed how to build your custom GraphQL API with Express.js.
Tell us about the projects you've created making use of GraphQL.
- Simple to create and manage My dashboard. My dashboard
- Support is available 24/7.
- The top Google Cloud Platform hardware and network is driven by Kubernetes to ensure maximum capacity
- Premium Cloudflare integration that improves speed as well as security
- Connect to the global audience by utilizing over 35 data centers and more than 275 POPs around the world.
The post first appeared on here
Article was posted on here