Create the URL shortener which is simple Utilizing Python (r) (r)
URL Shortener
Enter an https URL: Fill in the URL"/form>" Body>/html>" Body>/html>
This code generates an online form that has 2 labels and two input fields of input as well as one button.
The first input field dubbed URL
is the place where you write the URL you'd like to make use of, while in the second input field where you write the shorter URL.
In the address
input field has these attributes:
name
to identify the element ( e.g. URL)placeholder
to show the URL of an image- pattern: To determine the pattern of a URL which has the format https ://. *
is needed
for providing an input URL prior to sendingvalue
Go to the URL from which you've earlier utilized
In the second, there is a value attribute. It is referred to as value. It is a value attribute, that is used to find the attribute called new_url.. New_url is an simple URL.
is an uncomplicated URL created by the shorteners inside the Python Shorteners library, which is included in the main.py file (shown in the section below).
The entry form can be identified through the following picture:
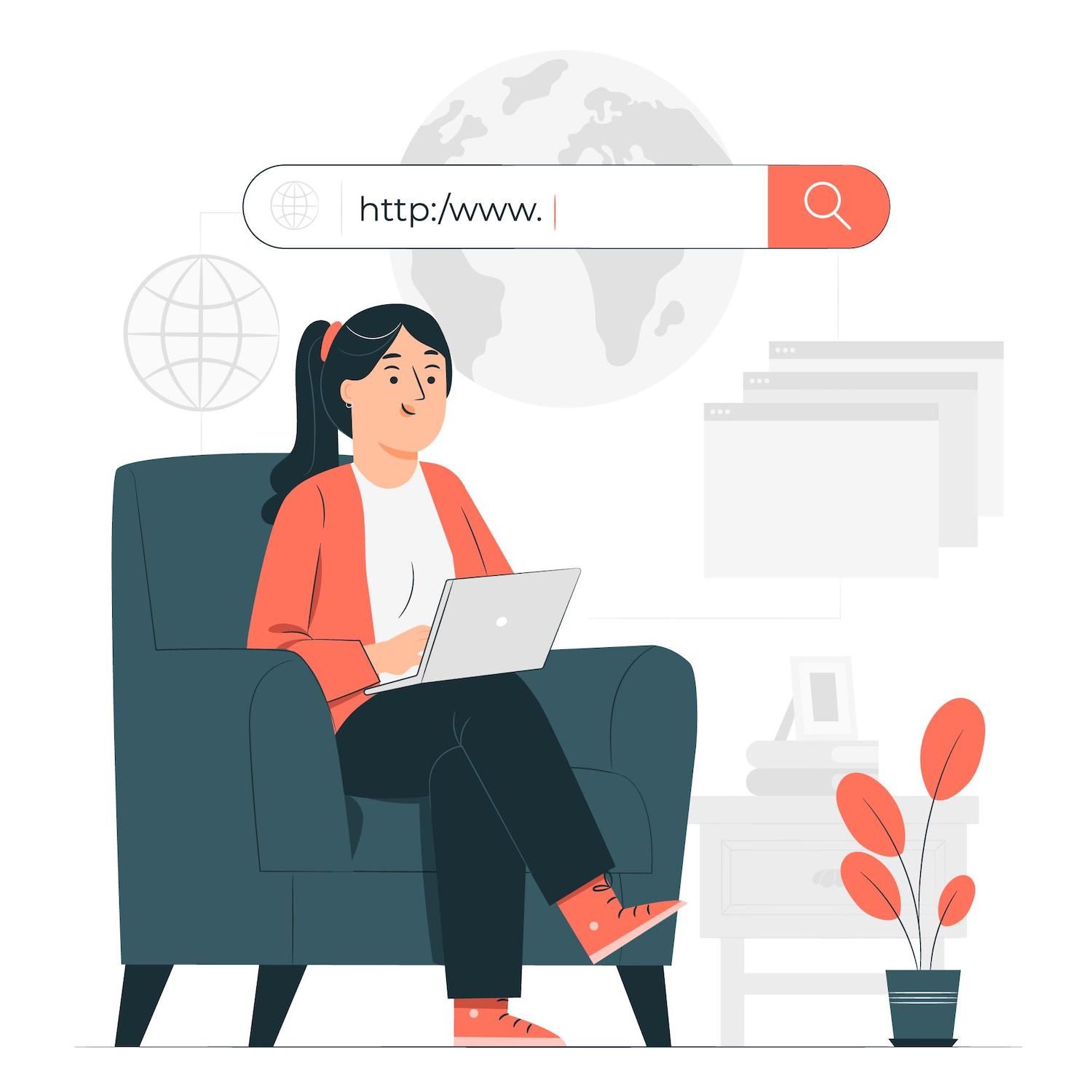
URL Shortening Code Using pyshorteners
When you've completed the form, you'll be able to edit the form in a more effective way by using Python as well as shorteners using Python.
The code converts the long URL into shorter URLs to run the web-based application. Go to your main.py file you created earlier. Add the following code section to save it. Save it in:
from flask import Flask, render_template, request import pyshorteners app = Flask(__name__) @app.route("/", methods=['POST', 'GET']) def home(): if request.method=="POST": url_received = request.form["url"] short_url = pyshorteners.Shortener().tinyurl.short(url_received) return render_template("form.html", new_url=short_url, old_url=url_received) else: return render_template('form.html') if __name__ == "__main__": app.run()
The above code loads the shorteners to include in the library pyshorteners library, as well as the following modules which are part of Flask. Flask framework. These are essential to minimize URLs
Flask
Flask Flask Framework was released previously.- render_template HTML0 render template HTML0 render template is a program for rendering which generates HTML documents. HTML documents are produced making use of directory templates.
template
. request
A class inside the Flask framework that holds all the data that the user transmits through the application's front end to the backend application like the HTTP request.
It then generates an application called home()
that takes URLs that are entered in the form and creates an abbreviated URL. It is possible to use app.route() as an app.route()
decorator to connect this function to the particular URL location to execute the application. The POST/GET methods deal with the requests.
Within"home ()", the "home()
function is comprised of an in between
and an expression which is constrained.
For the if
statement, if request.method=="POST"
, a variable called url_received
is set to request.form["url"]
, which is the URL submitted in the form. This is due to the reality that URL
is the name given to the input field that is specified in the HTML form. It was created in the past.
Then, a variable called short_url
is set to pyshorteners.Shortener().tinyurl.short(url_received)
. Two methods use the shorteners found in the pyshorteners library: .Shortener()
and .short()
. This .Shortener()
function allows you to build a version of the pyshorteners class. In addition, the .short()
function can be used to enter the URL for an input and to reduce the length of URL.
Short() function, also known as short() function, also known as brief()
function, tinyurl.short()
is among the shorteners that are vital part of the shorteners found in the pyshorteners shorteners libraries. These have many APIs. osdb.short()
is another API that could be utilized to accomplish similar tasks.
The render_template()
function is employed to generate form.html, the HTML templates form.html file. form.html and send URLs back to form with arguments. This function uses arguments. "new_url"
argument is now the argument short_url
and the argument for old_url
is now added the argument for old_url to the URL you are receiving
. The declaration's definition has been extended.
declaration's scope is increased beyond.
If you use the alternative
clause to discover how the technique that you use to make a request is different from POST the request will display using form.html HTML template. form.html HTML template will display.
A sample an example URL Shortener Web Application built with the help of Python Shorteners Library. Python Shorteners Library
To demonstrate the pyshorteners URL shortener application, navigate to the default route for the application, http://127.0.0.1:5000/, after running the application.
Enter a hyperlink you like below to the right of the web form:
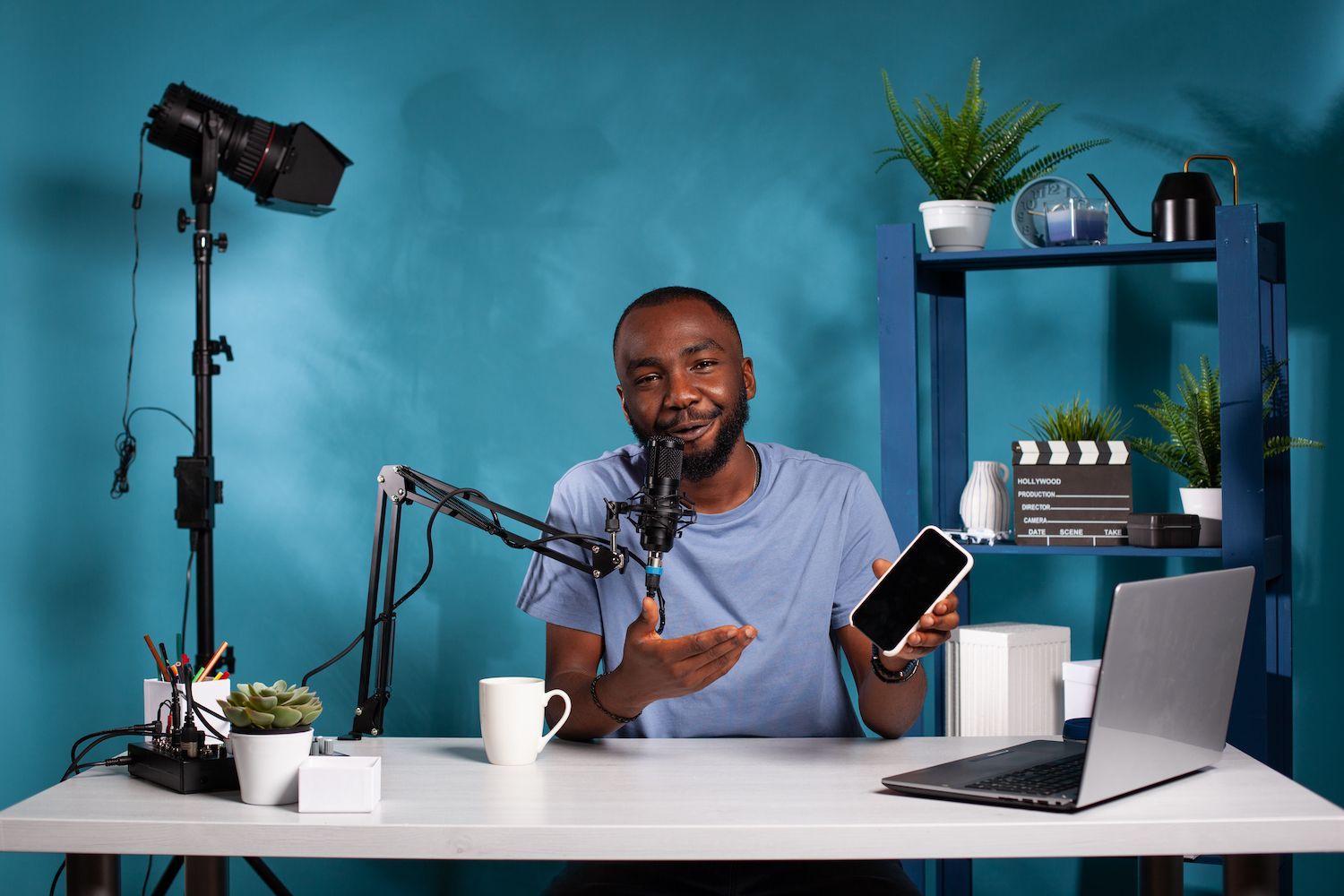
Hit on the link to send your message. Click the button to create URL. Select Submit to generate the URL as a version that is smaller. Make use of tinyurl
as the domain name when you click the box where it states the URL which was generated.
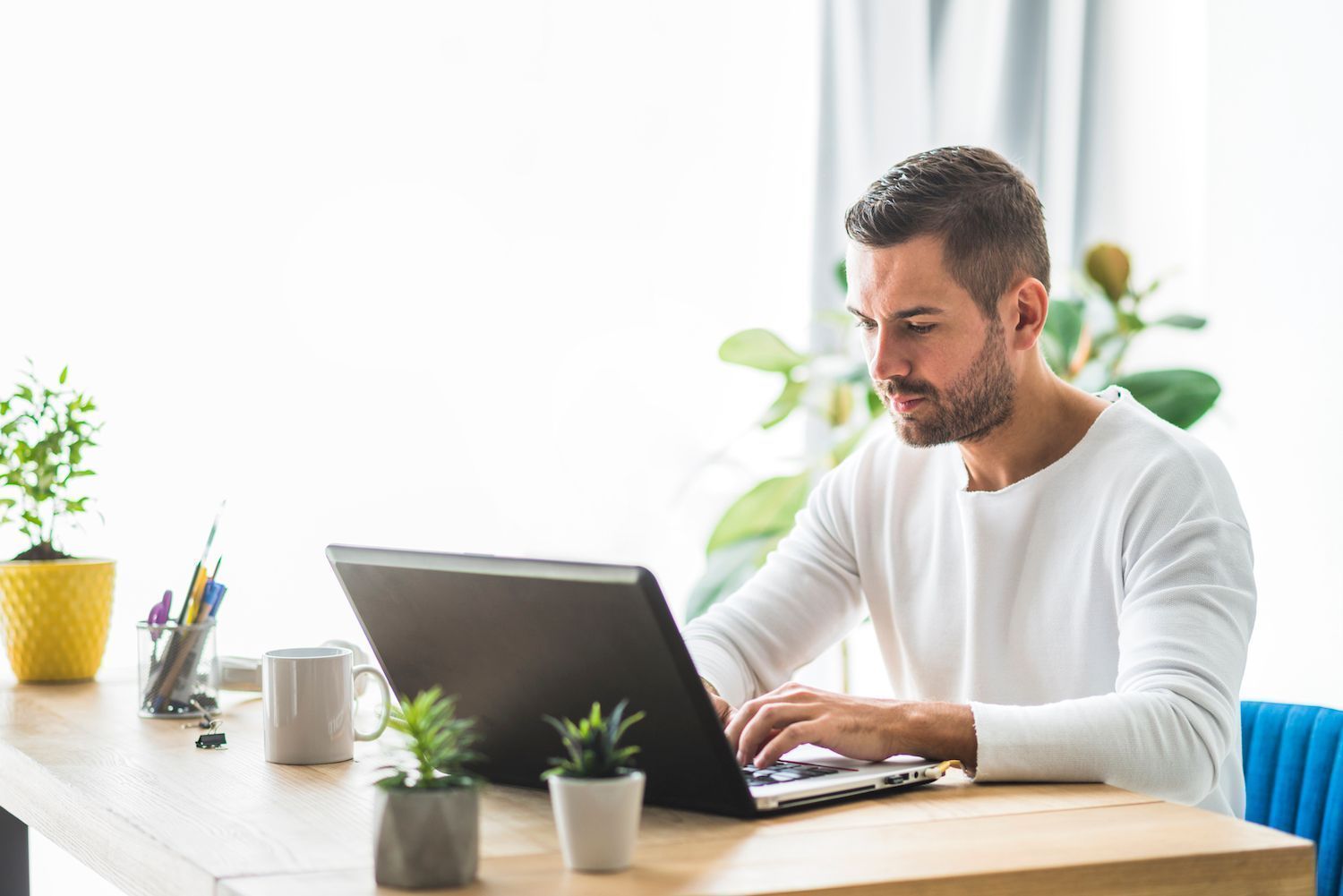
Utilizing Bitly API Module Bitly API Module is now in a position to create the URL Shortener App for use to shorten URLs across the Web. Application
In this class in this class, you'll build a URL shortening application using Bitly API. Bitly API. Bitly API. Bitly API module provides another method of shortening URLs. It also provides detailed information about clicks, the location of the user, and also the type of device that is used (such like a mobile device or desktop).
Create Bitly API. Bitly API. Install and download Bitly API. Install the Bitly API using the following instructions:
pip to install Bitly API-py3
Access tokens are required to gain allow access to Bitly API. Access tokens are required to access Bitly API. Access tokens are the access tokens that can be accessed when you log into Bitly..
When you've completed the sign-up process, you'll have the option of signing to sign up with Bitly. Once you've completed your signing-up procedure, sign in to Bitly to access your account dashboard.
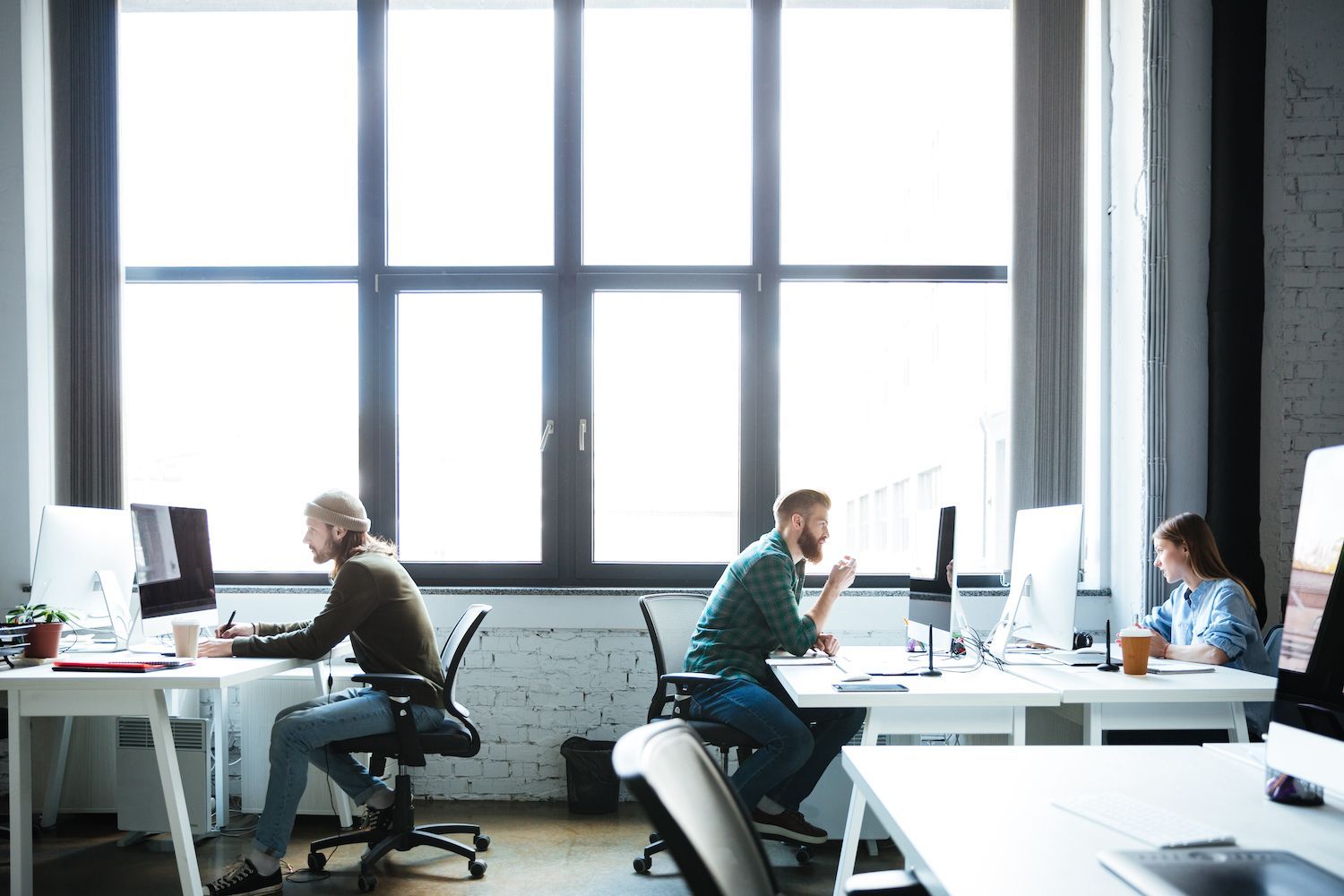
Click Settings in the sidebar to the left. After that, navigate sections within this API section, which is located inside the Developer Settings.
Create an access token through using the username that is entered in the space just above the token generator button. This is apparent in the following image. Keep the token in mind for later use in your applications:
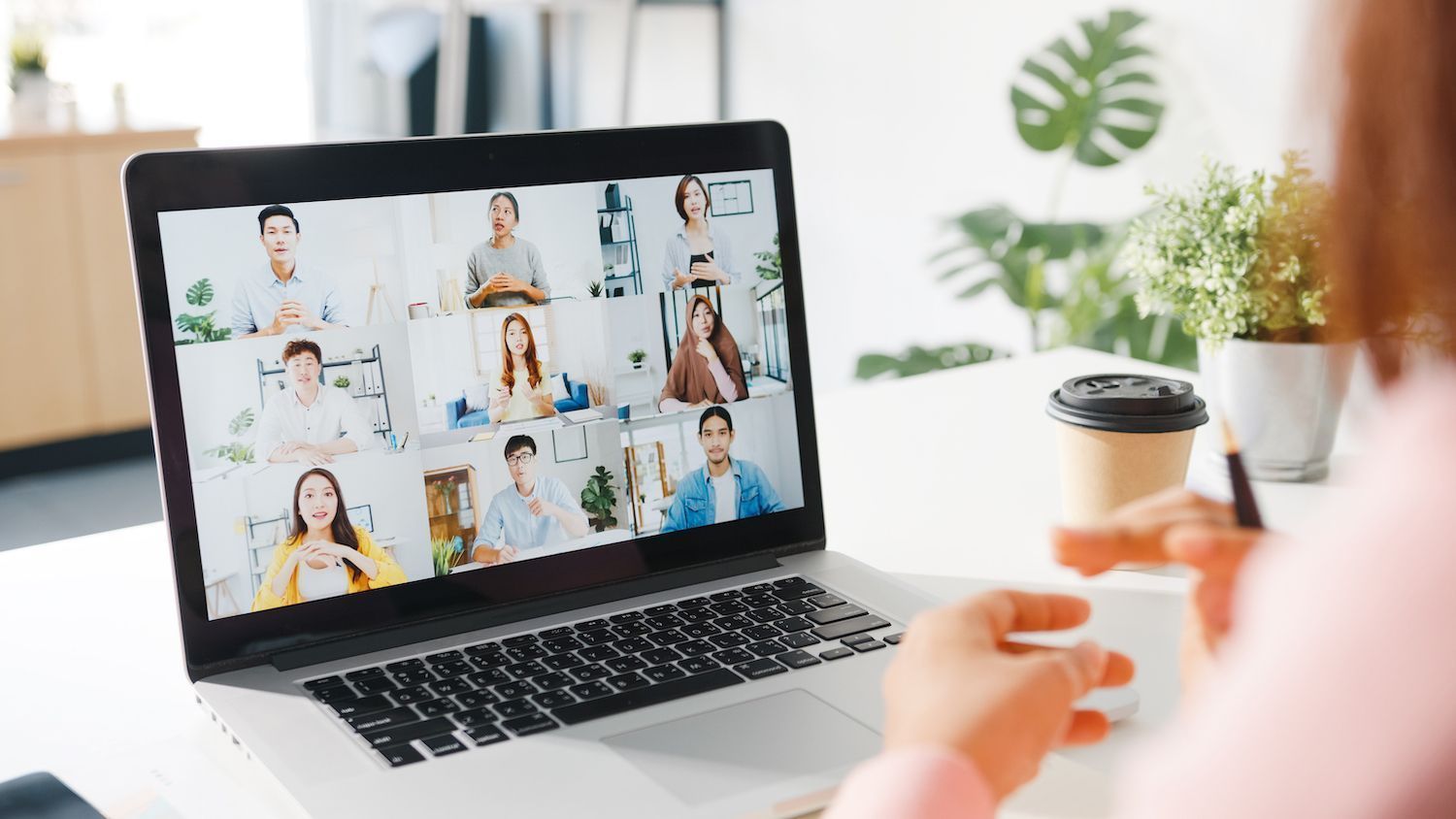
URL Shortening Code Using the Bitly API
After you have received the Bitly token has been received Bitly tokens are sent to Bitly It will then allow you to create a program for your site application to make your URL less long using Bitly API. Bitly API. Bitly API.
The same template you created to create the shorteners section, with minor modifications to the main.py file:
from flask import Flask, render_template, request import bitly_api app = Flask(__name__) bitly_access_token = "37b1xxxxxxxxxxxxxxxxxxxxxxxxxx" @app.route("/", methods=['POST', 'GET']) def home(): if request.method=="POST": url_received = request.form["url"] bitly = bitly_api.Connection(access_token=bitly_access_token) short_url = bitly.shorten(url_received) return render_template("form.html", new_url=short_url.get('url'), old_url=url_received) else: return render_template('form.html') if __name__ == "__main__": app.run()
In the case above, bitly_api
is loaded by using the import bitly_api
. The access token is then saved in a variable called bity_access_token
, as in bitly_access_token = "37b1xxxxxxxxxxxxxxxxxxxxxxxx"
.
"home"() function is a shortened version of URL. "home"()
function is a contraction of URL. It also includes"in-case-of-nothing" conditional expression. "in-case-of-nothing"
conditional expression.
When you are using this declaration, you should make use of the
option when POST is your preferred method of communication or your request follows the same format as POST.
The URL you enter in the form will be changed in accordance with the the requested URL
variable.
The bitly_api.Connection(access_token=bitly_access_token)
function connects to the Bitly API and passes it the access token you saved earlier as an argument.
In order to reduce the amount of URLs it is possible to utilize using the bitly.shorten() function. bitly.shorten()
function. It is a method to cut down the size of websites by providing the URL_received
value as an argument, then storing the results in an array, which is known as the short_url
.
The form then renders and then URLs are returned to display on the form. The rendering procedure is accomplished using the rendering templates()
function. When you've submitted your request form, that will be the case when this
it becomes the most important element of the form.
If you select the other
clause of the assertion, then the model will render using rendering_template() function. render_template()
function.
Examples for URL Shortener Web Application built by Bitly API. Bitly API
To demonstrate the Bitly API URL shortener application, navigate to the default route for the application, http://127.0.0.1:5000/, after running the application.
Input a favourite URL in the box on the right side of the form for websites:
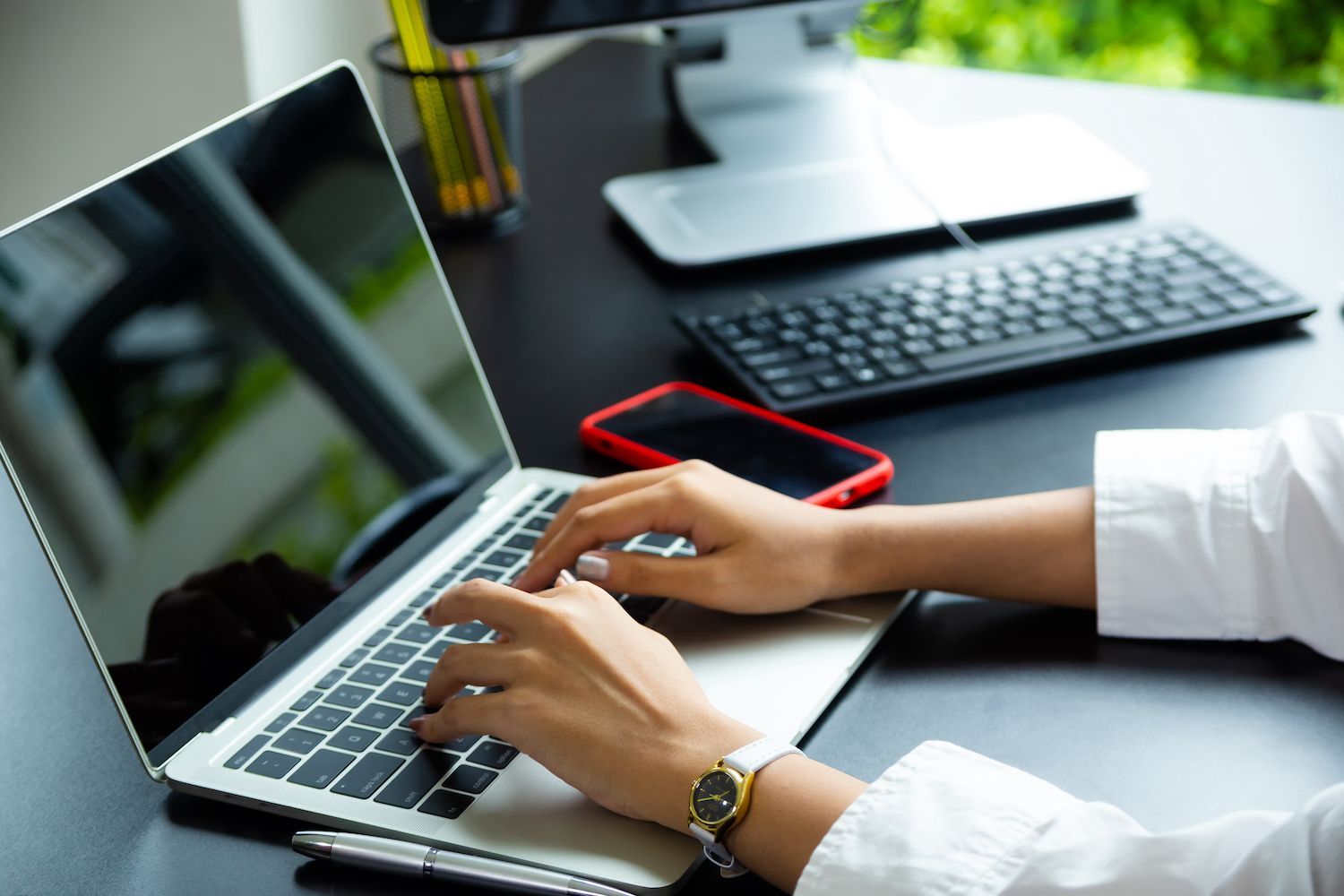
Select "Submit" to create a URL using bit.ly
as the domain. The other part of the process is the website application
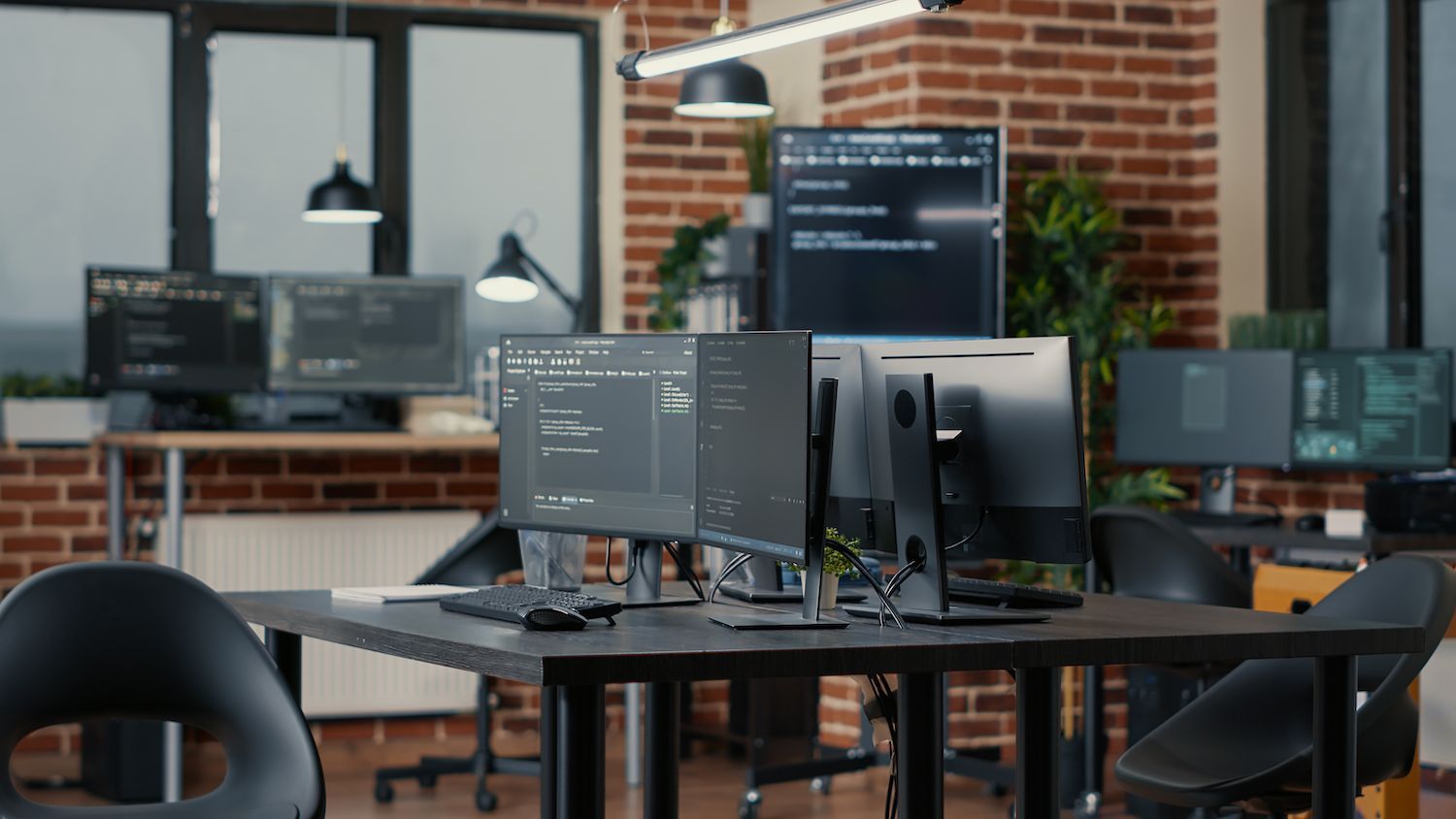
Utilizing Bitly API to use the Bitly API to use the Bitly API to shorten URLs in your Python program is as easy to accomplish as it sounds.
Summary
URL shorteners offer brief URLs that people are able to use and are more attractive and require less space. In this article, you'll find information about URL shorteners, as well the benefits they offer and methods to create an URL shortener-related web application with Python using Pyshorteners and the Bitly API. The Python shorteners library allows for shorter URLs. Bitly API provides detailed analytics. Bitly API gives precise analytics and shorter URLs.
Adarsh Chimnani
Adarsh is a web-designer (MERN stack) He is a lover of design that is related to gaming (Unity3D) and an avid fan of anime. Adarsh is amazed by the information he gathers through his curiosity. He also applies the knowledge he's acquired in the workplace as well as disseminating the knowledge to others.
The post first appeared here. this website
The article was first published on this website.
This post was first seen on here
Article was first seen on here