A Comprehensive Guide To Laravel Authentication
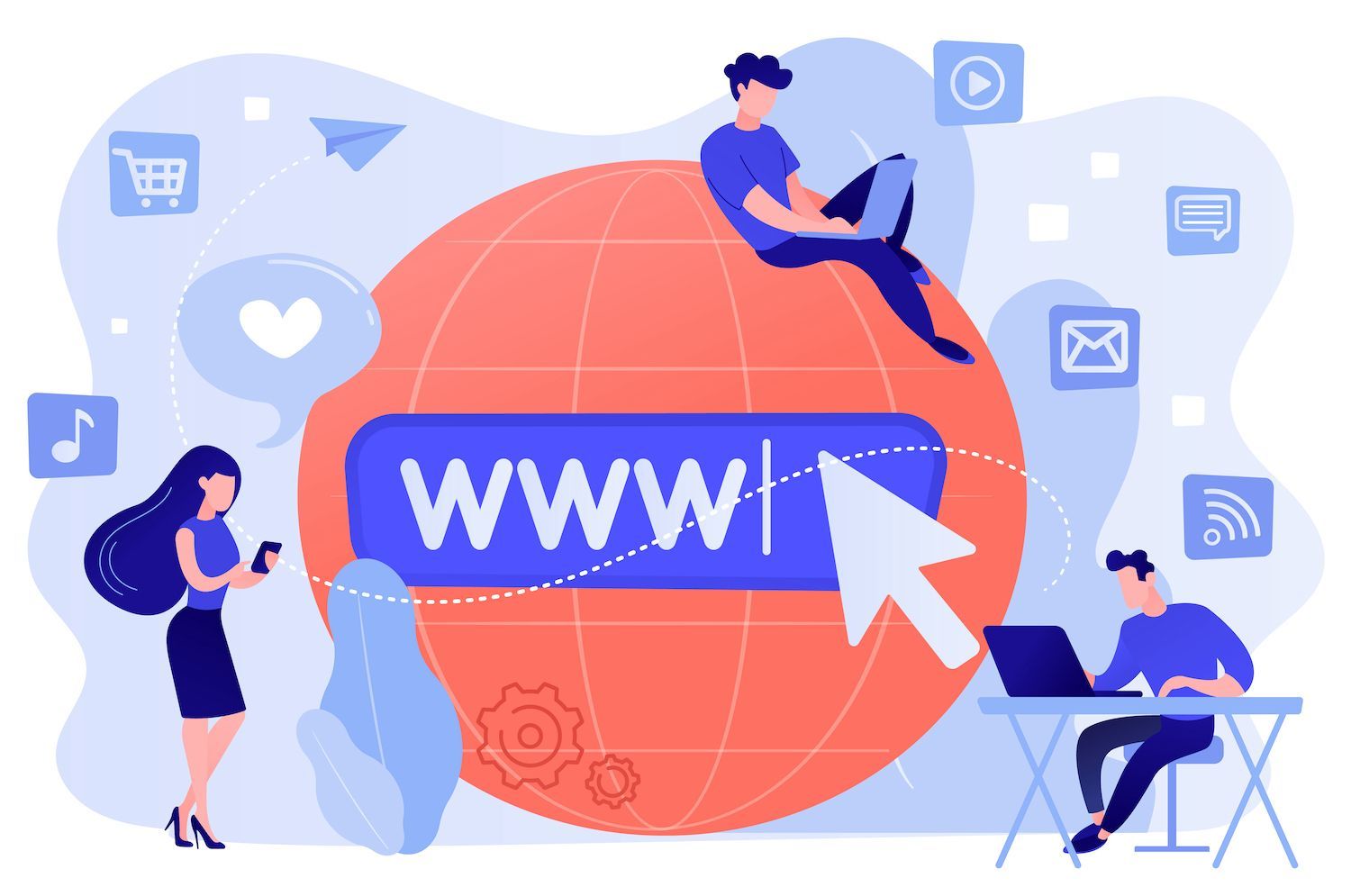
An authentic authentication feature is among web application's crucial and fundamental capabilities. Web frameworks like Laravel provide a range of options for users to log in.
You can implement Laravel authentication features swiftly and securely. However, using these features in the wrong way could lead to danger as malicious individuals could exploit them.
This article will help you get to be aware of before beginning with your preferred Laravel authentication techniques.
Check it out!
An Intro To Laravel Authentication
Our authentication settings are defined in a file titled config/auth.php
. This file provides a range of options to tweak and alter the authentication behaviour of Laravel.
The first step is to determine the defaults for authentication. The option you select controls the application's standard security "guard" and password reset options. The default settings are able to be changed as required, but they're a perfect beginning for most apps.
Next, you define authentication guards for your application. Here, our default configuration makes use of sessions storage along with Eloquent's user provider. Each authentication driver comes with a user provider.
return [ /* Defining Authentication Defaults */ 'defaults' => [ 'guard' => 'web', 'passwords' => 'users', ], /* Defining Authentication Guards Supported: "session" */ 'guards' => [ 'web' => [ 'driver' => 'session', 'provider' => 'users', ], ], /* Defining User Providers Supported: "database", "eloquent" */ 'providers' => [ 'users' => [ 'driver' => 'eloquent', 'model' => App\Models\User::class, ], // 'users' => [ // 'driver' => 'database', // 'table' => 'users', // ], ], /* Defining Password Resetting */ 'passwords' => [ 'users' => [ 'provider' => 'users', 'table' => 'password_resets', 'expire' => 60, 'throttle' => 60, ], ], /* Defining Password Confirmation Timeout */ 'password_timeout' => 10800, ];
Then, we ensure that each authentication device has the user's provider. It defines how users are retrieved from your database or other methods of storage for preserving the user's data. You can configure different sources for each model table, when you own more than one model or user tables. These sources may be assigned to different security guards that you've defined.
Users may also want to change their passwords. If this is the case, you can set different password reset parameters if you have multiple tables or model within the application that you want to use different settings that are based on particular user type. The expiration period is the time period for which every reset token is in use. This function ensures that tokens are short-lived, so they are less likely to be guessed. This can be changed when needed.
It is your responsibility to determine when a password confirmation times out in which case the user is required to input their password once more on the screen to confirm. The default duration of timeout is 3 hours.
The various kinds of Laravel Authentication Methods
Password Based Authentication
A basic way to authenticate the user's identity, it's widely used by thousands of companies, however with how technology is evolving, the method is becoming outdated.
Vendors are required to enforce sophisticated password implementations and ensure that users experience minimal inconvenience.
It's quite simple it is as simple as entering the name and the password, and, in the event that inside the Database there is found that there is a match between these two items, the server chooses to check the information and allow users access the resources for a specific amount of time.
Token-Based Authentication
The method is employed to issue the user an individual token upon verification.
By using this token, the user have access to relevant sources. The privilege is in place up to the date that expires the token.
If the token is activated it does not need to enter a username or password. However, after retrieving a fresh token, both are needed.
Tokens are frequently used in a variety of scenarios since they're non-stateless entities which contain all the authentication data.
Offering a method to distinguish the difference between token generation and verification allows vendors to enjoy a wide deal of freedom.
Multi-Factor Authentication
The term "authority" refers to the notion that is to utilize at minimum two methods of authentication, increasing the level of security that it offers.
This is generally done using an encryption method, and then users are provided with an identification number to use to their cell phone. Businesses that use this method are advised to look out for false positives and network outages that could cause serious issues when expanding quickly.
What do I need to do to Implement the Laravel Authentication
Manual Authentication
Starting with registering users and creating routes within routes/web.php
.
We will create two routes for you to look at the form , and another for signing up:
use App\Http\Contrllers\Auth\RegisterController; use Illuminate\Support\Facades\Route; /* Web Routes Register web routes for your app's RouteServiceProvider in a group containing the "web" middleware */ Route::get('/register', [RegisterController::class], 'create']); Route::post('/register', [RegisterController::class], 'store']);
and create the control needed to accomplish these things:
php artisan make controller Auth/RegisterController -r
Update the code now using the following method:
namespace App\Http\Controllers\Auth; use App\Http\Controllers\Controller; use illuminate\Htpp\Request; class RegisterController extends Controller public function create() return view('auth.register'); public function store(Request $request)
The controller is empty , and returns the view you are able to join. It is possible to create the view inside the view/resources/auth
and then name it register.blade.php. register.blade.php
.
Once everything is set now, it is time to go on our or register
route, and then fill out the form below:
public function store(Request $request) $credentials = $request->only('email', 'password'); if (Auth::attempt($credentials, $request->filled('remember'))) $request->session()->regenerate(); return redirect()->intended('/'); return back()->withErrors([ 'email' => 'The provided credentials do not match our records. ', ]);
Now, we have the form which a user can complete and get all the necessary information. Then, collect the users' data then validate the data and then put it into the database to make sure the accuracy of all data. That's why you have use a transaction in your database to verify that the information that you input is correct.
We'll use Laravel's request validation tool to make sure that all three required credentials are present. It is imperative to ensure that your password matches the format and format. Also, it's unique in the users
database. The password is verified and contains the minimum number of characters:
namespace App\Http\Controllers\Auth; use App\Http\Controllers\Controller; use Illuminate\Foundation\Auth\User; use Illuminate\Http\Request; use Illuminate\Support\Facades\Hash; class RegisterController extends Controller public function store(Request $request) confirmed public function create() return view('auth.register');
When our input has been checked, any input that is against the validation process will cause an error. The error will be displayed in the formof:
When we've made an account for a user using the Store
method, we want to sign in into the user's account. There are two methods in the way we can accomplish this. It is possible to do the task manually or with this technique using facade of the Auth interface.
When a user signs into this system, they shouldn't take them back at that Register screen. Instead, they must be directed to a different page, like a homepage or dashboard. We'll do that to do this:
namespace App\Http\Controllers\Auth; use App\Http\Controllers\Controller; use App\Providers\RouteServiceProvider; use Illuminate\Foundation\Auth\User; use Illuminate\Http\Request; use Illuminate\Support\Facades\Auth; use Illuminate\Support\Facades\Hash; class RegisterController extends Controller public function store(Request $request) confirmed public function create() return view('auth.register');
And now that we are registered users and logged in
and with the -n password us, we have to make sure that he is able to safely sign out.
Laravel suggests we shut down the session and then create a new token of security upon the logout. That's exactly what we are going to accomplish. First, we need to develop a completely different logout
route , using LogoutController's destruction
method:
use App\Http\Controllers\Auth\RegisterController; use App\Http\Controllers\Auth\LogoutController; use Illuminate\Support\Facades\Route; /* Web Routes Here is where you can register web routes for your application. These routes are loaded by the RrouteServiceProvider with a group which contains the "web" middleware group. Make something truly amazing! */ Route::get('/register', [RegisterController::class, 'create']); Route::post('/register', ['RegisterController::class, 'store']); Route::post('/logout', [Logoutcontroller::class, 'destroy']) ->middleware('auth');
The process of logging out via"auth," the "auth" middleware application is vital. It is essential that the user cannot use the service if they're not signed in.
Then, you can create an account similar to the one we created earlier:
php artisan make:controller Auth/LogoutController -r
You can ensure that the request is taken as a part of the destruction
method. The user is signed out with an Auth facade. We then disable the session, and then regenerate the token and redirect users to the home page:
namespace App\Http\Controllers\Auth; use App\Http\Controllers\Controller; use Illuminate\Http\Request; use Illuminate\Support\Facades\Auth; class LogoutController extends Controller public function destroy(Request $request) Auth::logout(); $request->session()->invalidate(); $request->session()->regenerateToken(); return redirect('/');
Remembering Users
The majority, if not all modern websites offer that "remember me" checkbox after you log into their form.
If we would like to add to the "remember me" feature, we can use a boolean as an additional argument for the"try" method.
When the token appears to be valid, Laravel will keep the user authenticated for a lengthy time or until they are manually signed out. The table of users must contain the strings forget_token
(this is the reason why that we create tokens) column. This is where we'll keep the "remember me" token.
The default migration is already available to users.
For starters, you'll have add to begin, the "Remember Me" field :
After that, you will be able to obtain your credentials through the request and then apply them to the try technique of the Auth facade.
If the user's name can be known, we'll log him in and redirect the user to our home page. If not, we'll throw an error.
public function store(Request $request) { $credentials = $request->only('email', 'password'); if (Auth::attempt($credentials, $request->filled('remember'))) $request->session()->regenerate(); return redirect()->intended('/'); return back()->withErrors([ 'email' =>
Resetting Passwords
The majority of web-based applications allow users to reset their passwords.
There will be another method to reset the password that was forgotten and then design this controller in the exact manner as we did. Also, we'll develop a brand new way to reset password buttons, that will contain the necessary token to be used through the whole procedure.
Route::post('/forgot-password', [ForgotPasswordLinkController::class, 'store']); Route::post('/forgot-password/token', [ForgotPasswordController::class, 'reset']);
Within the store option, we will take the email address provided in the request and confirm the email address in exactly the same way that we do in normal situations.
After this, we can apply SendResetLink, a SendResetLink
method , which is available via the password interface.
In response, we'd like to update the status if it succeeded in sending the link or if there is any other issue:
namespace App\Http\Controllers\Auth; use App\Http\Controllers\Controller; use Illuminate\Http\Request; use Illuminate\Support\Facades\Password; class ForgotPasswordLinkController extends Controller public function store(Request $request) $request->validate([ 'email' => 'required
After the reset link is sent to the user's email It is our responsibility to take care of the procedure following that.
The token will be sent to you and email address along with the new password after you send the email address and then verify it.
After this, we're capable of applying to reset the password with the password façade to allow Laravel manage everything else in the background.
It is always a good option to create a password hash in order to secure it.
Then, we will check whether the password has been reset, and if it was, then we send users to login screens and display it being a message "Success. In the event that it was not reset, we display an error indicating that the password could not be reset:
namespace App\Http\Controllers\Auth; use App\Http\Controllers\Controller; use Illuminate\Http\Request; use Illuminate\Support\Facades\Hash; use Illuminate\Support\Facades\Password; use Illuminate\Support\Str; class ForgotPasswordController extends Controller public function store(Request $request) $request->validate([ 'token' => 'required', 'email' => 'required
Laravel Breeze
Laravel Breeze is an easy solution to Laravel authentication functions, such as registration and login features for password reset, login authentication, and password verification. The Breeze can be used to add authentication to the brand-new Laravel application.
Installation and Installation and
After creating your Laravel application All you need do is to set up your database, then perform the migrations and run the laravel/breeze app through composer:
composer require laravel/breeze -dev
Then, follow these steps:
php artisan breeze:install
The system will then publish your authentication views and routes as well as controllers and other resources it makes use of. After this step you will fully control everything Breeze gives you. Breeze provides you with.
We now need to render our frontend application, so we will install our JS dependencies (which utilize @vite):
Install NPM
:
npm run dev
Once you have logged in after logging in, your login and registration hyperlinks must appear on your homepage, and everything should work well.
Laravel Jetstream
Laravel Jetstream enhances Laravel Breeze by introducing useful functions as well as additional frontend stacks.
It provides the ability to sign in, register, and email verification. Additionally, it provides Two-factor security as well as session control. API support via Sanctum as well as team administration.
There is a choice between Livewire or Inertia at the frontend when installing Jetstream. Backends use Laravel Fortify which is a frontend agnostic, "headless" authentication backend used by Laravel.
Installation and Installation
We'll install it via the composer for our Laravel Project:
composer require laravel/jetstream
Following this, we'll use the jetstream install php jetstream]
command, which accepts stackas
choices such as Livewire
or Inertia
. You can then use the option to select the -team
option to enable the feature for teams.
This will also install Pest PHP in order to testing purposes.
Finally, we need to develop the front end for the application using these methods:
npm install npm run dev
Laravel Fortify
Laravel Fortify is a backend authentication system that is frontend-independent. It's not necessary to utilize Laravel Fortify to make use of the Laravel authentication functions.
Fortify can also be used to start tools like Breeze in addition to Jetstream. Fortify can run Fortify as a standalone application that acts as an implementation of the backend. If you choose to use it standalone application your frontend needs to utilize Fortify's routing routes to access them.
Installation and Set-up
Fortify is a program that we can install Fortify via composer:
composer require laravel/fortify
The next step is to make available Fortify's sources:
php artisan vendor:publish -provider="Laravel\Fortify\FortifyServiceProvider"
After this, we will create a new app/Actions directory in addition to the new FortifyServiceProvider, configuration file, and database migrations.
Finally, run:
php artisan migrate
Or:
php artisan migrate:fresh
And then the Fortify is now ready to be ready to go.
Laravel Socialite
Installation
It is possible to download it through composer:
composer require laravel/socialite
Configuration and use
After installing it, we have to add the appropriate credentials to the OAuth vendor that our application depends upon. The credentials will be added to config/services.php for each service.
The configuration will have to check your key to previously used services. The keys that you can compare include:
- Twitter (For OAuth 1.0)
- Twitter-Oauth-2 (For OAuth 2.0)
- Github
- Gitlab
- bitbucket
A service configuration could be in the following manner:
'google' => [ 'client_id' => env("GOOGLE_CLIENT_ID"), 'client_secret' => env("GOOGLE_CLIENT_SECRET"), 'redirect' => "http://example.com/callback-url", ],
Authenticating Users
In order to accomplish this, there are two paths, one that will redirect the user's request to OAuth service providers.
use Laravel\Socialite\Facades\Sociliate; Route::get('/auth/redirect', function () return Socialite:;driver('google')->redirect(); );
And one for the return call to the provider upon authenticating:
use Laravel\Socialite\Facades\Socialite; Route:;get('/auht/callback', function () $user = Socialite:;driver('google')->user(); // Getting the user data $user->token; );
Socialite provides the redirect method in conjunction with the facade, which redirects the user to the OAuth provider. The user method analyzes the request and then retrieves the data about the user.
When we receive the user's information, we need to confirm that it is there on our databases. If it is, we must authenticate it. If it does not exist there is no database there, we'll have to create an entry that represents the person:
use App\Models\User; use Illuminate\Support\Facades\Auth; use Laravel\Socialite\Facades\Socialite; Route::get('/auth/callback', function () /* Get the user */ $googleUser = Socialite::driver('google')->user(); /* Create the user if it does not exist Update the user if it exists Check for google_id in database */ $user = User::updateOrCreate([ 'google_id' => $googleUser->id, ], [ 'name' => $googleUser->name, 'email' => $googleUser->email, 'google_token' => $googleUser->token, 'google_refresh_token' => $googleUser->refreshToken, ]); /* Authenticates the user using the Auth facade */ Auth::login($user); return redirect('/dashboard'); );
If we want to restrict the access of users to certain areas, we could apply this scoping
method. We apply to the request for authentication. It will combine all the previously mentioned scopes and assign them to the one that is specific to.
Another alternative is to employ this method: The setScopes
method, which replaces any scope that is already in use:
use Laravel\Socialite\Facades\Socialite; return Socialite::driver('google') ->scopes(['read:user', 'write:user', 'public_repo']) ->redirect(); return Socialite::driver('google') ->setScopes(['read:user', 'public_repo') ->redirect();
We have all the information about how to contact the number of the user after the callback. Let's have time to look over some of the info we can get from it.
OAuth1 user has token
as well as tokenSecret
:
$user = Socialite::driver('google')->user(); $token = $user->token; $tokenSecret = $user->tokenSecret;
OAuth2 provides you with the token
, refreshToken
as well as expires in
:
$user = Socialite::driver('google')->user(); $token = $user->token; $refreshToken = $user->refreshToken; $expiresIn = $user->expiresIn;
Both OAuth1 and OAuth2 support getID GetNickname, getName getEmail, and getAvatar.
$user = Socialite::driver('google')->user(); $user->getId(); $user->getNickName(); $user->getName(); $user->getEmail(); $user->getAvatar();
And if we want to get user details from a token (OAuth 2) or a token and secret (OAuth 1), sanctum provides two methods for this: userFromToken
and userFromTokenAndSecret
:
use Laravel\Socialite\Facades\Socialite; $user = Socialite:;driver('google')->userFromToken($token); $user = Socialite::driver('twitter')->userFromTokenAndSecret($token, $secret);
Laravel Sanctum
Laravel Sanctum is a light authenticator that's ideal for SPAs (Single Page Applications) and mobile apps. It allows users to create multiple API tokens that have specific scopes. This defines the scopes of actions that can be performed by a token.
Uses
Sanctum can be used to provide API tokens for the user without the complexities associated with OAuth. These tokens usually have lengthy durations, much like the many years. They are however easily changed and can be canceled by customers at any point.
Installation and installation and
It is possible to set it up using composer
composer require laravel/sanctum
It is also necessary to create the files for configuration and migration:
php artisan vendor:publish -provider="Laravel\Sanctum\SanctumServiceProvider"
Once we've made new documents for the migration, we are ready to transfer the following files:
php artisan migrate or php artisan migrate:fresh
What is the best way to issue API tokens
Before issuing tokens, our User model should use the Laravel\Sanctum\HasApiTokens trait:
use Laravel\Sanctum\HasApiTokens; class User extends Authenticable use HasApiTokens;
When we have the user, we can issue a token by calling the createToken
method, which returns a Laravel\Sanctum\NewAccessToken instance.
You may use to use the PlainTextToken
method in the NewAccessToken instance to display the the SHA-256 the text in plain form in the token.
Tips and best practices to authenticating Laravel
Validating Sessions on Different Devices
As we have discussed previously, invalidating the session is vital after a user logs out However, the session should be accessible to every device belonging to the user.
This option is typically used when the user changes or changes their password in order to delete their account on another device.
Utilizing the Auth facade It's a straightforward process. Since the technique is being used comes with auth
along with auth.session middleware auth.session middleware
, we can employ this technique to shut down other devices
using the static method provided by the facade
Route::get('/logout', [LogoutController::class, 'invoke']) ->middleware(['auth', 'auth.session']);
use Illuminate\Support\Facades\Auth; Auth::logoutOtherDevices($password);
Configuration With Author: Routes()
The route method of the Auth facade acts as a helper to generate each route needed to allow authenticating users.
The options are Login (Get Post or Find), Logout (Post), Register (Get Post, Get) and Password Change/Email (Get Post, Get).
If you are able to call the procedure to the façade, this can accomplish:
public static fucntion routes(array $options = []) if (!static::$app->providerIsLoaded(UiServiceProvider::class)) throw new RuntimeException('In order to use the Auth:;routes() method, please install the laravel/ui package. '); static::$app->make('router')->auth($options);
We're curious to know what happens when we use the static method called on the router. This is a tricky job due to the nature of how facades operate, but the method called is like the following:
/** Register the usual methods of authentication for an application. @param array $options @return void */ public function auth(array $options = []) // Authentication Routes... $this->get('login', 'Auth\[email protected]')->name('login'); $this->post('login', 'Auth\[email protected]'); $this->post('logout', 'Auth\[email protected]')->name('logout'); // Registration Routes... if ($options['register'] ?? true) $this->get('register', 'Auth\[email protected]')->name('register'); $this->post('register', 'Auth\[email protected]'); // Password Reset Routes... if ($options['reset'] ?? true) $this->resetPassword(); // Email Verification Routes... if ($options['verify'] ?? false) $this->emailVerification();
It generates by default all the routes, excluding the authentication email route. It will always generate the Login as well as Logout routes. The other routes we can control via the selection choice.
If we want to permit users to login and logout but not sign in, then we can use these arrays of options:
$options = ["register" => true, "reset" => false, "verify" => false];
Security Routes, Custom Guards, and Protecting Routes
We need to ensure that the routes we use can be accessible only to authenticated users and is easily accomplished through either calling the middleware method on the front of Route or by using the middleware method in the context of it.
Route::middleware('auth')->get('/user', function (Request $request) return $request->user(); ); Route::get('/user', function (Request $request) return $request->user(); )->middleware('auth');
This security measure ensures every request that is received will be authenticated.
Password Confirmation
In order to ensure website security, you often want to confirm the password of an individual before moving on with the next job.
It is important to determine an appropriate path starting through the view of confirm password in order to process the request. It will verify and send the user to their final destination. In addition we ensure that the password we use is verified during the time frame of the session. In default, the password is confirmed every 3 hours. It is possible to alter the frequency through modifying the configuration file in config/auth.php:
use Illuminate\Http\Request; use Illuminate\Support\Facades\Hash; use Illuminate\Support\Facades\Redirect; Route::post('/confirm-password', function (Request $request) if (!Hash::check($request->password, $request->user()->password)) return back()->withErrors([ 'password' => ['The provided password does not match our records.'] ]); $request->session()->passwordConfirmed(); return redirect()->intended(); )->middleware(['auth']);
Authenticable Contract
The Authenticable contract located at Illuminate\Contracts\Auth defines a blueprint of what the UserProvider facade should implement:
namespace Illuminate\Contracts\Auth; interface Authenticable public function getAuthIdentifierName(); public function getAuthIdentifier(); public function getAuthPassord(); public function getRememberToken(); public function setRememberToken($value); public function getrememberTokenName();
The interface allows the system of authentication to interact alongside every "user" class which implements it.
This is true regardless of the ORM or storage layers are used. By default, Laravel has the App\Models\User which implements this interface which can be seen in the configuration files:
return [ 'providers' => [ 'users' => [ 'driver' => 'eloquent', 'model' => App\Models\User::class, ], ], ];
Authentication Events
There are plenty of events that occur during the authentication process.
Depending on your goals, you can attach listeners to those events in your EventServiceProvider
.
Rapidly create new users
The process of making a new user quickly can be done by using the application user:
$user = new App\User(); $user->password = Hash::make('strong_password'); $user->email = '[email protected]'; $user->name = 'Username'; $user->save();
or via or through the static creation method that is available or through the interface of the user:
User::create([ 'password' => Hash::make('strong-password'), 'email' => '[email protected]', 'name' => 'username' ]);
Summary
The Laravel ecosystem includes a variety of basic kits to get your application functioning that utilize an authentication system, such as Breeze or Jetstream. The kits are highly customizable because the code is developed by us as well as we are able to modify how we'd like to use it as a blueprint should we need to.
There are numerous security concerns with regards to authentication, and the complexities involved. However each of them can be resolved with ease using the tools Laravel can provide. They can be easily customizable and easy to use.
- Simple setup and management of My Dashboard. My dashboard
- 24/7 expert support
- The most effective Google Cloud Platform hardware and network powered by Kubernetes for the highest capacity
- A business-level Cloudflare integration, which improves performance and security.
- Reaching a global audience with up to 35 data centers, and more than 275 PoPs spread across the world
Article was posted on here